この記事ではAngularの『ngFor ディレクティブ』について、
- ngFor ディレクティブとは
- ngFor ディレクティブの構文
- ngFor ディレクティブを用いたプログラム例
- ngFor ディレクティブで使用できる特殊変数
などを図を用いて分かりやすく説明するように心掛けています。ご参考になれば幸いです。
ngFor ディレクティブとは
ngFor ディレクティブは、JavaScriptのfor...of命令に相当するディレクティブです。
ngFor
ディレクティブはAngularの組み込みディレクティブの1つであり、指定された配列から各要素を順に取り出し、その内容をループ処理させることができます。
ngFor
ディレクティブを用いることで、動的なリストやテーブルを簡単に描画させることができるようになります。
ngFor ディレクティブの構文
ngFor
ディレクティブの構文を以下に示します。
ngFor ディレクティブの構文
<任意の要素 *ngFor="let 仮変数 of 処理対象の配列">繰り返したいコンテンツ</任意の要素>
ngFor ディレクティブを用いたプログラム例
ngFor
ディレクティブを用いたプログラム例を以下に示します。
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<ul>
<li *ngFor="let item of items">
{{ item.id }}: {{ item.name }}: {{ item.color }}
</li>
</ul>
`,
})
export class AppComponent {
items = [
{ id: 1, name: 'りんご', color: '赤' },
{ id: 2, name: 'バナナ', color: '黄' },
{ id: 3, name: 'ぶどう', color: '紫' },
{ id: 4, name: 'メロン', color: '緑' },
];
}
実行結果
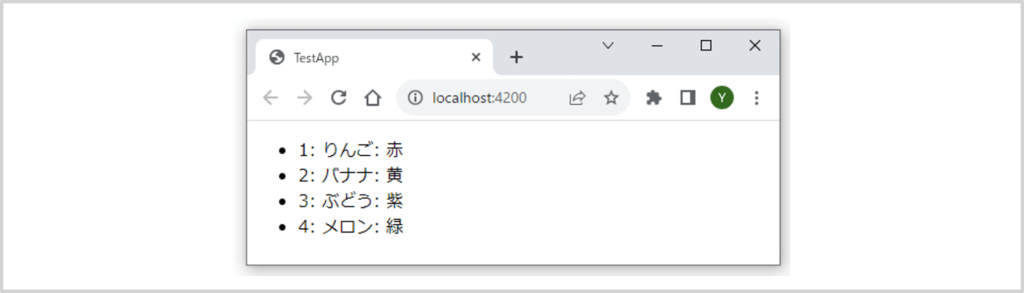
上記のプログラムでは、あらかじめ用意されたオブジェクトの配列items
をngFor
ディレクティブを用いて表示させています。オブジェクトの配列items
の要素を順に仮変数item
に代入し、その内容を表示させています。
ngFor ディレクティブで使用できる特殊変数
ngFor
ディレクティブは下表に用いる特殊変数を用いることで、ループに関わる情報にアクセスできます。
例えば、配列のインデックスを取得したり、配列の各要素が「最初の要素」か「最後の要素」かなどを確認できるようになります。
変数 | 概要 |
index | 要素のインデックス値 |
first | 最初の要素であればtrue 、そうでなければfalse |
last | 最後の要素であればtrue 、そうでなければfalse |
even | インデックス値が偶数であればtrue 、そうでなければfalse |
odd | インデックス値が奇数であればtrue 、そうでなければfalse |
ngFor
ディレクティブで使用できる特殊変数を用いたプログラム例を以下に示します。
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<table border="1">
<thead>
<tr>
<th>インデックス</th>
<th>配列の各要素の値</th>
<th>first(最初の要素か)</th>
<th>last(最後の要素か)</th>
<th>even(要素のインデックス値が偶数か)</th>
<th>odd(要素のインデックス値が奇数か)</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of items; index as i; first as isFirst; last as isLast; even as isEven; odd as isOdd">
<td>{{ i }}</td>
<td>{{ item }}</td>
<td>{{ isFirst ? '〇' : '-' }}</td>
<td>{{ isLast ? '〇' : '-' }}</td>
<td>{{ isEven ? '〇' : '-' }}</td>
<td>{{ isOdd ? '〇' : '-' }}</td>
</tr>
</tbody>
</table>
`,
})
export class AppComponent {
items = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I'];
}
実行結果
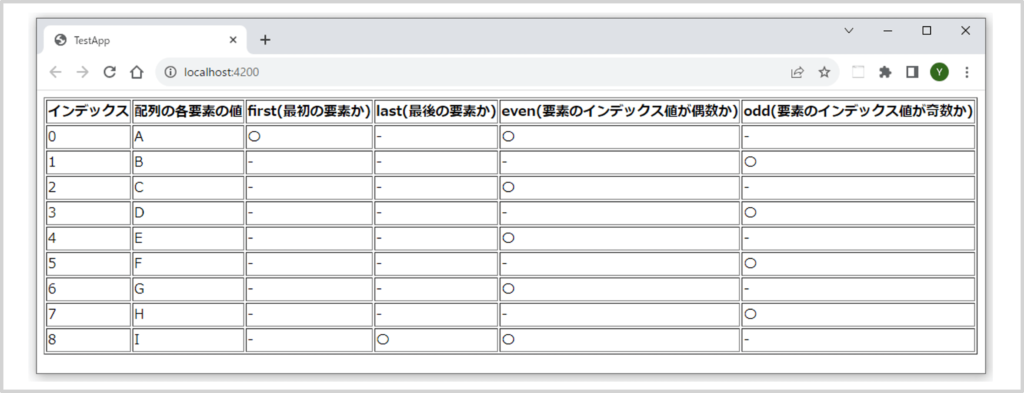
特殊変数をngFor
ディレクティブでループするコンテンツで利用する場合には、ngFor
に対して「index as i
」のようにローカル変数を割り当てておかなければいけない点に注意してください。上記のプログラムでは、「index as i
」と記述することで、特殊変数index
をローカル変数i
で利用できるようにしています。
また、「index as i
」ではなく「let i = index
」と書いてもOKです。つまり、以下の様にプログラムを置き換えても構いません。
<tr *ngFor="let item of items; let i = index; let isFirst = first; let isLast = last; let isEven = even; let isOdd = odd">
特殊変数を用いてスタイルを変える
特殊変数を用いることで、配列の最初と最後だけスタイルを変えたり、偶数だけスタイルを変えたりすることもできます。
以下のプログラムは、先ほど説明したプログラム例に対して、以下のスタイルを追加している一例です。
- 最初と最後の行に背景色を付ける。
- 偶数行の文字色を青にする。
以下のプログラムでは、[class.xxx]="condition"
の形式を用いて、条件がtrue
の時に指定したクラスを要素に適用しています。そして、styles
プロパティ内でそのクラスのスタイルを定義しています。
app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<table border="1">
<thead>
<tr>
<th>インデックス</th>
<th>配列の各要素の値</th>
<th>first(最初の要素か)</th>
<th>last(最後の要素か)</th>
<th>even(要素のインデックス値が偶数か)</th>
<th>odd(要素のインデックス値が奇数か)</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let item of items; index as i; first as isFirst; last as isLast; even as isEven; odd as isOdd"
[class.first-row]="isFirst" [class.last-row]="isLast" [class.even-row]="isEven">
<td>{{ i }}</td>
<td>{{ item }}</td>
<td>{{ isFirst ? '〇' : '-' }}</td>
<td>{{ isLast ? '〇' : '-' }}</td>
<td>{{ isEven ? '〇' : '-' }}</td>
<td>{{ isOdd ? '〇' : '-' }}</td>
</tr>
</tbody>
</table>
`,
styles: [
`
.first-row {
background-color: #e0f7fa;
}
.last-row {
background-color: #fce4ec;
}
.even-row {
color: blue;
}
`,
],
})
export class AppComponent {
items = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I'];
}
実行結果
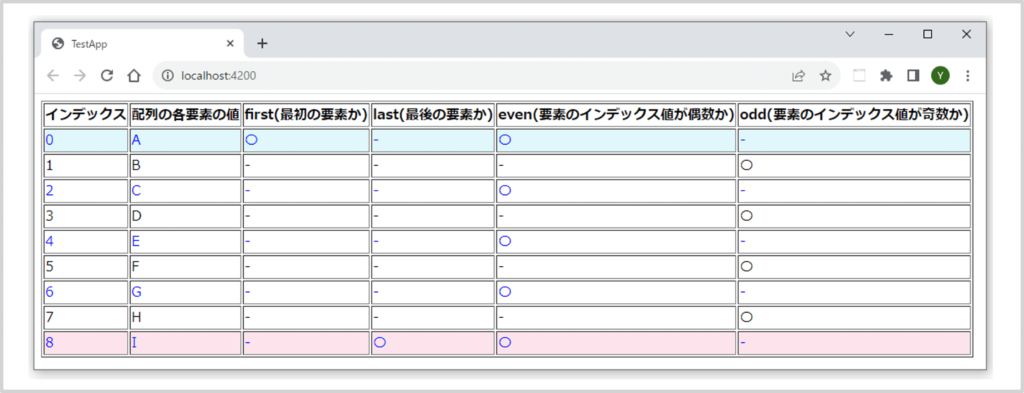
上記のプログラム例において、[class.xxx]="condition"
を用いずにngClass
を用いた場合は以下のようになります。ngClass
を使用することで、複数のクラスを条件付きで適用することができます。
以下のプログラムでは、[ngClass]
ディレクティブにオブジェクトを渡しています。このオブジェクトのキーはクラス名、値は真偽値の条件となります。値がtrue
の場合、そのキーのクラスが要素に適用されます。
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<table border="1">
<thead>
<tr>
<th>インデックス</th>
<th>配列の各要素の値</th>
<th>first(最初の要素か)</th>
<th>last(最後の要素か)</th>
<th>even(要素のインデックス値が偶数か)</th>
<th>odd(要素のインデックス値が奇数か)</th>
</tr>
</thead>
<tbody>
<tr
*ngFor="let item of items; index as i; first as isFirst; last as isLast; even as isEven; odd as isOdd"
[ngClass]="{
'first-row': isFirst,
'last-row': isLast,
'even-row': isEven
}"
>
<td>{{ i }}</td>
<td>{{ item }}</td>
<td>{{ isFirst ? '〇' : '-' }}</td>
<td>{{ isLast ? '〇' : '-' }}</td>
<td>{{ isEven ? '〇' : '-' }}</td>
<td>{{ isOdd ? '〇' : '-' }}</td>
</tr>
</tbody>
</table>
`,
styles: [
`
.first-row {
background-color: #e0f7fa;
}
.last-row {
background-color: #fce4ec;
}
.even-row {
color: blue;
}
`,
],
})
export class AppComponent {
items = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I'];
}
本記事のまとめ
この記事ではAngularの『ngFor ディレクティブ』について、以下の内容を説明しました。
- ngFor ディレクティブとは
- ngFor ディレクティブの構文
- ngFor ディレクティブを用いたプログラム例
- ngFor ディレクティブで使用できる特殊変数
お読み頂きありがとうございました。